Importing Code Coverage Results in the NDepend Code Model
- Why importing coverage results files?
- How to specify coverage results files to import in NDepend project?
- How to specify coverage results files to import in Azure DevOps / TFS extension?
- Exclusion of code elements from coverage statistics with an attribute
- Exclusion of code elements from coverage statistics with a .runsettings file
Make the most of your code coverage data with NDepend (2 minutes)
Why importing coverage results files?
Here are the benefits of importing code coverage at NDepend analysis time:
The global coverage percentage is shown in the NDepend dashboard. Also coverage results is compared against the baseline coverage results.
- Trend charts can be obtained for coverage data in the report and in the NDepend UI.
See it live here.
- Several default code rules are about validating code coverage like: make sure that all sufficiently complex methods are 100% covered. It is easy to write your own rules.
- Several default quality gates are about enforcing code coverage like: require 80% of code coverage on new classes added since the baseline or fail the build if the criteria is not achieved. It is easy to write your own quality gate.
Coverage green/red highlights can be shown in the web report. See it live here.
Coverage ratio is shown for each source file in the report. The grid can be sorted by coverage ratio. See it live here.
- Harness search facilities to explore the coverage data.
- Query code coverage through Code Query Linq
-
Visualize the code coverage data through colored treemaping in the metric view.
For example the screenshot below shows code coverage by tests on the NDepend code base.
How to specify coverage results files to import in NDepend project?
Code coverage data is imported from coverage result files generated by the coverage tools.
Coverage results files can be specified from NDepend > Project Properties > Analysis > Code Coverage.
In this dialog you can also specify a directory. NDepend will attempt to find one or several coverage files in this directory at analysis time.
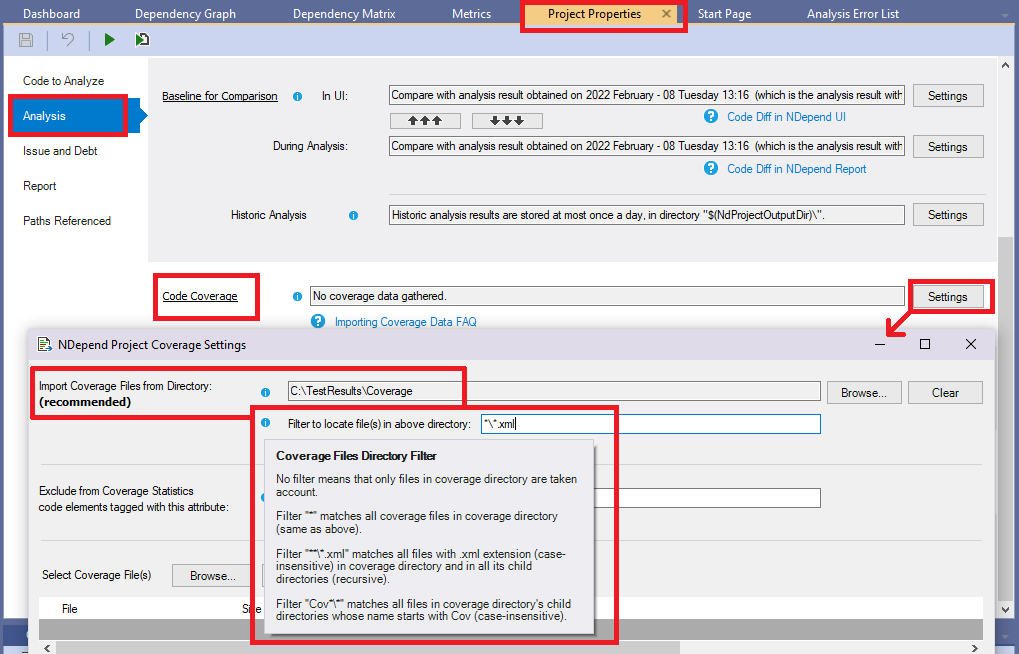
As shown by the screenshot above, since NDepend version 2022.1 it is possible to provide a wildcard-based filter to precisely locate the coverage file under the directory specified.
Code coverage result files imported might be generated by different tools. In such situation NDepend will merge data properly.
How to specify coverage results files to import in Azure DevOps / TFS extension?
If you have a test task with Code Coverage Enabled, NDepend analysis will automatically import the resulting code coverage. Results will be significantly more useful with coverage imported.
However if you are running a specific or custom task to gather code coverage with a technology different than Microsoft Visual Studio coverage you can still use the Get Coverage Files from a specified directory NDepend task option. NDepend will be smart enough to determine for each coverage file (there can be one or many), which coverage technology has been used to generate it and how to import this data.
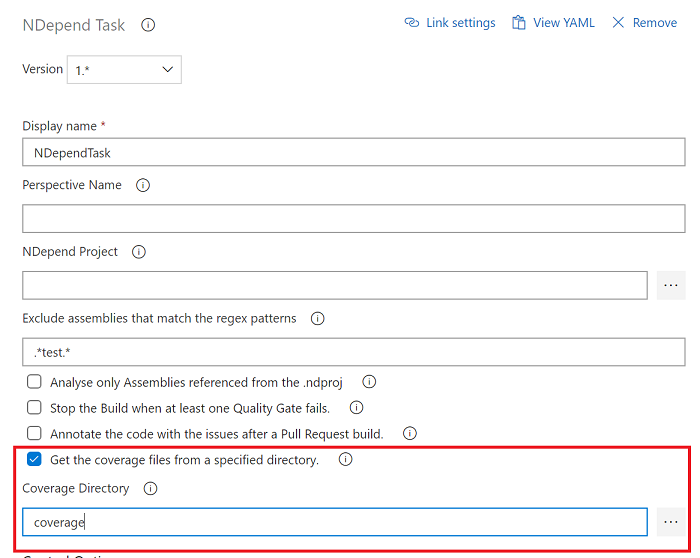
Exclusion of code elements from coverage statistics with an attribute
All methods and types tagged with System.Diagnostics.CodeAnalysis.ExcludeFromCodeCoverageAttribute will be excluded from NDepend coverage statistics, no matter the coverage technology used.
This attribute System.Diagnostics.CodeAnalysis.ExcludeFromCodeCoverageAttribute can be applied at assembly level but not in a C# file this way :
[assembly: System.Diagnostics.CodeAnalysis.ExcludeFromCodeCoverage]
The right way to declare this attribute at the assembly level is to insert this section in the .csproj project file (this tip only works on .NET Core, 5, 6 ... projects, not on .NET Framework projects):
<Project Sdk="Microsoft.NET.Sdk"> <PropertyGroup> <TargetFramework>net6.0</TargetFramework> <ImplicitUsings>enable</ImplicitUsings> <Nullable>enable</Nullable> </PropertyGroup> <ItemGroup> <AssemblyAttribute Include="System.Diagnostics.CodeAnalysis.ExcludeFromCodeCoverage" /> </ItemGroup> </Project>
All methods and types tagged with System.Diagnostics.DebuggerNonUserCodeAttribute or System.Diagnostics.DebuggerHiddenAttribute will be excluded from NDepend coverage statistics only for Visual Studio coverage files.
In VisualNDepend menu: Project Properties > Analysis > Code Coverage > Settings you can provide your own exclusion attribute. See the screenshot in the previous section, there YourNamespace.ExclusionAttribute is used for example.
In such case you need to specify both the full namespace qualifier and the Attribute suffix.
Notice that with Code Query Linq you can query which assembly, namespace, type or method is excluded explicitly (through an attribute or a .runsettings file, see below) with the property: ICodeContainer.ExcludedFromCoverageStatistics.
from m in Application.Methods
where m.ExcludedFromCoverageStatistics
select m
This is a subset of assembly, namespace, type or method whose code coverage data is not available (that can be queried through the property ICodeContainer.CoverageDataAvailable).
from m in Application.Methods
where !m.CoverageDataAvailable
select m
Exclusion of code elements from coverage statistics with a .runsettings file
XML formatted file with extension .runsettings can be used to specify coverage exclusion and inclusion rules. These rules are based on regular-expressions. For more details, please refer to the official Microsoft documentation concerning coverage exclusion through .runsettings file.
NDepend can read and process such .runsettings file's coverage exclusion and inclusion rules to obtain coverage statistics similar to the ones provided by other coverage tools. A .runsettings file can be provided from Project Properties > Analysis > Code Coverage > Settings:
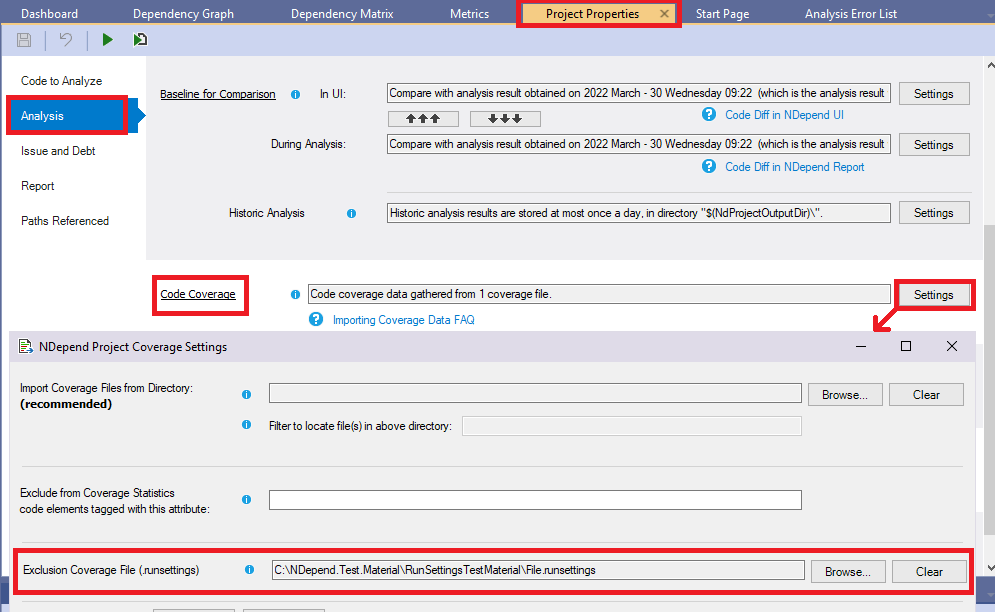
When analyzing an NDepend project that references a .runsettings file, some NDepend analysis logs provide details about the processing of these coverage exclusion and inclusion rules.
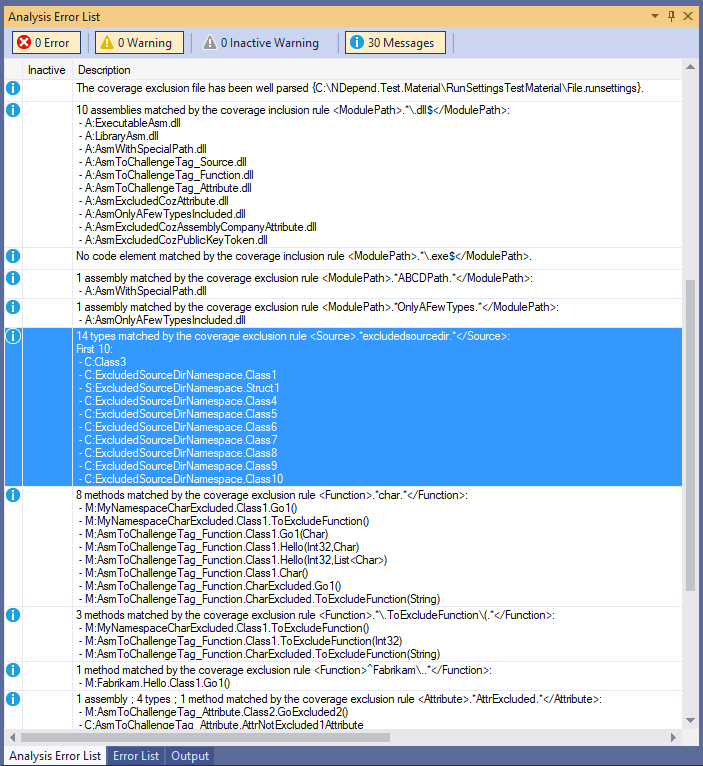
Keep in mind that the Exclude section (in a .runsettings file) takes precedence over the Include section: if an assembly, a type or a method is listed in both Include and Exclude, it will not be included in code coverage. The same way if a method or a type is listed by an Include section but its parent assembly is listed by an Exclude section, it will not be included in code coverage.
Visual Studio 2022, 2019, 2017, 2015, 2013, 2012 and 2010 Code Coverage
NDepend supports both Visual Studio coverage file formats:
- The binary format, file extension .coverage. This format is only readable from Windows, not from Linux or MacOS. The reason is that the DLL provided by Microsoft to read this format is compiled against .NET Framework and cannot be executed on Linux or MacOS.
-
The XML format, file extension .coveragexml since Visual Studio 2010, and .xml for previous versions. This format can be read on all OS.
Only the XML format with <CoverageDSPriv> root element is supported.
An other less common XML format exists with a <results> root element and same extension .coveragexml. This other format is not supported.
.NET Core coverage requires <DebugType>Full</DebugType> to be set both on unit test projects and tested projects.
.coverage result files can be obtained with the tool VSTest.Console.exe with the /EnableCodeCoverage option, for example:
"%programfiles(x86)%\Microsoft Visual Studio\2017\Enterprise\Common7\IDE\CommonExtensions\Microsoft\TestWindow\VSTest.Console.exe" ^ "..\bin\netcoreapp2.1\Tests1.dll" ^ "..\bin\netcoreapp2.1\Tests2.dll" ^ /EnableCodeCoverage /InIsolation
You can manually export XML coverage files from binary coverage files from Visual Studio with this option:
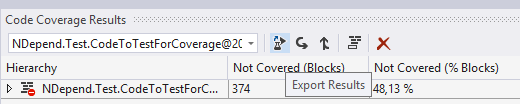
To obtain code coverage from within the NDepend Azure DevOps / TFS extension, just tick the Code Coverage Enabled option in the Test task.
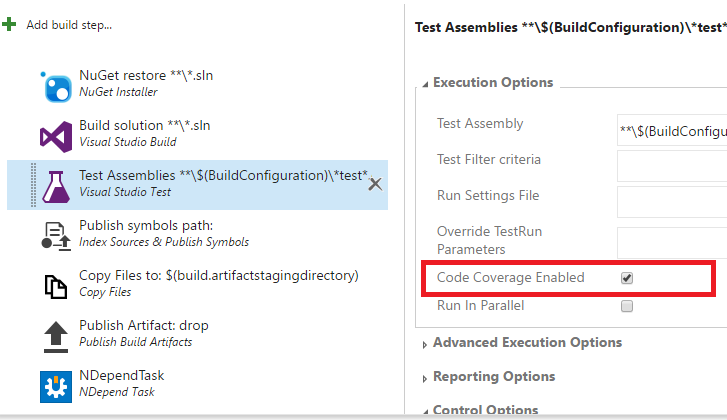
OpenCover
Just reference OpenCover xml coverage files created with OpenCover.Console.exe, or reference a directory that contains these files.
Typically OpenCover.Console.exe will be invoked this way to obtain OpenCover xml coverage files.
"%LOCALAPPDATA%\Apps\OpenCover\OpenCover.Console.exe" ^ -output:"%CD%\OpenCoverDateFile.xml" ^ -register:user ^ -target:"%VSINSTALLDIR%\Common7\IDE\CommonExtensions\Microsoft\TestWindow\vstest.console.exe" ^ -targetargs:"UnitTestProject1\bin\Debug\UnitTestProject1.dll"
AltCover
AltCover outputs coverage XML with the OpenCover format. As explained above, NDepend can read OpenCover format.
Here is a tutorial to get coverage files from AltCover.
dotnet test /p:AltCover=true
Coverlet
Coverlet can output its result in OpenCover format. Coverage results are then stored in a file named coverage.coverlet.xml. As explained above, NDepend can read OpenCover format.
dotnet test myapp.tests/myapp.tests.csproj /p:CollectCoverage=true /p:CoverletOutputFormat=opencover
Cobertura
Coverlet can also output its result in Cobertura XML format. Since NDepend version 2024.2.1 this format is supported to import coverage data.
Note: Avoid the Cobertura format, originating from the Java ecosystem, because it captures coverage data only at the line level. It is less accurate than other .NET coverage formats already supported by NDepend. This lack of precision is illustrated in this screenshot of source file coverage in the NDepend report:
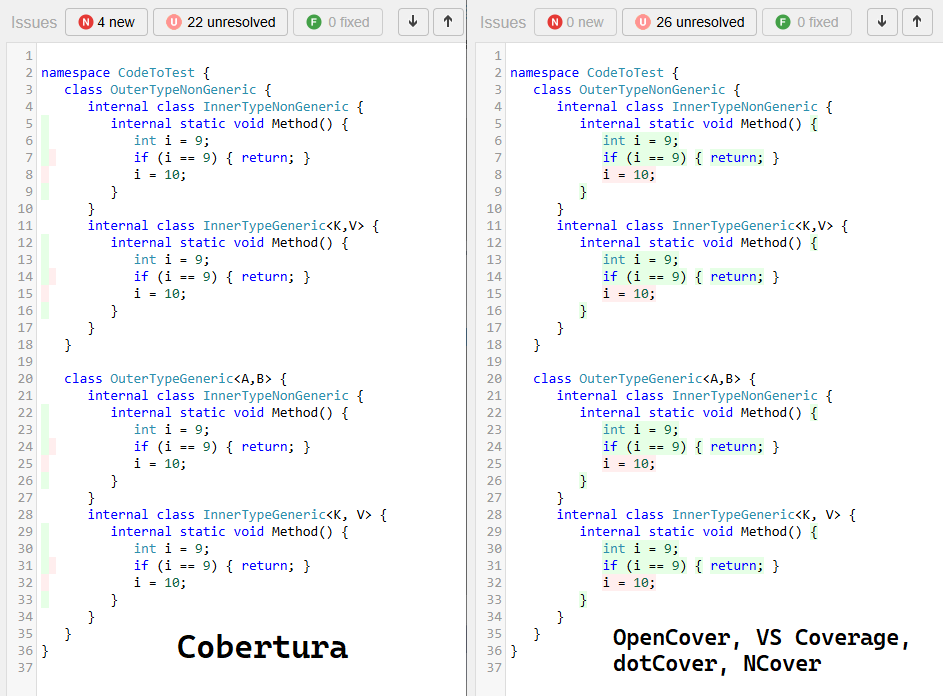
NCrunch 4.5.0 and above
Since NCrunch version 4.5.0 released in November 2020, NCrunch can export coverage data to OpenCover format XML files.
NDepend can import coverage data from OpenCover format XML files as explained in the section above.
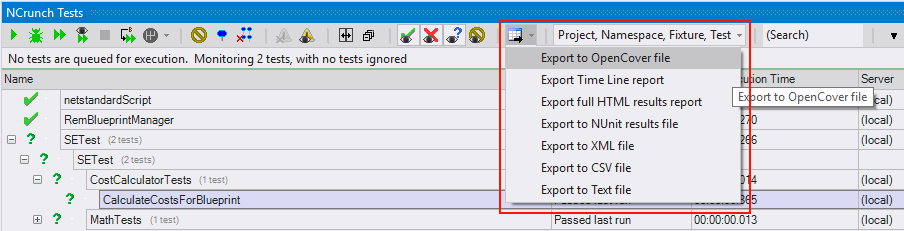
JetBrains DotCover
The dotCover console runner is a command-line tool distributed free of charge. Free dotCover console runner can be obtain from the Jetbrains website here. |
Since NDepend version 2023.2, the tool can parse the dotCover's ReportType DetailedXml. This report type let's show coverage green/red highlights in report source file. The report type can be defined this way in your DotCover configuration file (usually named coverage.xml):
<?xml version="1.0" encoding="utf-8"?><AnalyseParams>
<Executable>C:\App\Bin\MyApp.exe</Executable>
<Output>..\DotCoverCoverage.xml</Output>
<ReportType>DetailedXml</ReportType>
</AnalyseParams>
Then just run:
dotCover.exe cover C:\App\coverage.xml
You can precise also report type DetailedXml when exporting from the Jetbrain tooling UI.
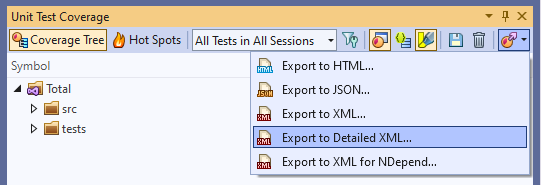
Note that the export option Export to XML for NDepend doesn't contain source green/red highlights and was dedicated for NDepend version 2023.1 and lower.
See also this article about using TeamCity, DotCover and NDepend, by Laurent Kempe:
Using TeamCity integrated DotCover coverage files with NDependNCover 3.X and above
NDepend reads NCover v3 XML format, hence if you are using NCover v4 or above, you need to export your coverage file with v3 format as explained in this NCover documentation. This looks like :
ncover export --project="MyProject" --execution="3/12/2017" --file="C:\Users\Support\Desktop\Report\coverage-export" --format=v3xml
If you are using TestDriven.NET and/or NCoverExplorer.exe you can access folder that contains coverage file from the Explore Coverage Folder menu:
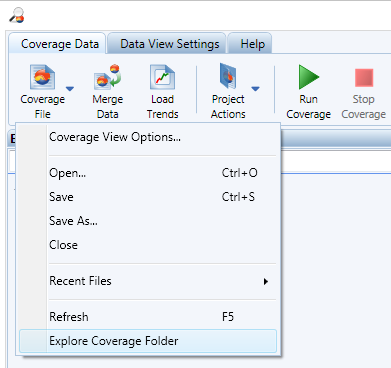